solving captchas
solving captcha programatically is a pain. me and this other dude at work took about two weeks to solve version 2 of google recaptcha which we learned later, was an enterprise versioned captcha. i did not understand what that meant, until i read between the lines of the docs.
this blog is a simple sidenote on how to solve recaptcha (v2) with 2captcha API. you can read the docs, which btw, is amazing.
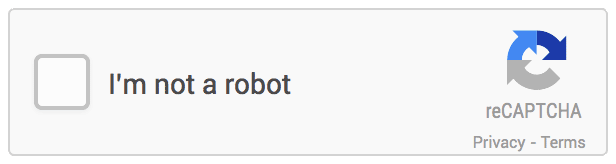
prequisities
- 2captcha API key
- install requests
step 1
right click around the captcha area –> inspect element
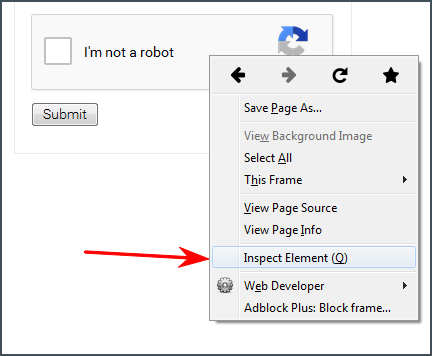
step 2
find the “data-sitekey” parameter and copy the value. this will be the ‘googlekey’ value.
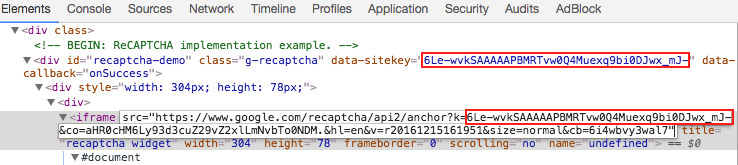
step 3
send a http GET request to submit a captcha.
import requests #pip install requests
API_KEY = '1abc234de56fab7c89012d3'
URL = 'http://2captcha.com/in.php'
SITE = 'https://site-with-captcha.com'
response = requests.get(URL,
params = {
'key': API_KEY,
'method': 'userrecaptcha',
'googlekey': 'key-from-step2',
'pageurl' : SITE
})
step 4
if you came this far, you will get the ID of your captcha as plain text, like:
OK|2122988149
if you get an error message, do not proceed to the next step. refer the documentation if you get any errors.
step 5
make a 15-20 seconds timeout and then, submit another GET request:
URL = 'http://2captcha.com/res.php'
response = requests.get(URL,
params = {
'key':API_KEY,
'action': get,
'id': 2122988149
})
if captcha is already solved, server will respond with a randomly generated token. if it does not, you can repeat your request in 5 seconds.
step 6
in developer’s console, find the textarea with id=“g-recaptcha-response”, and send the received code.

i used selenium to send the code back like so:
driver.execute_script("""
document.getElementById(
"g-recaptcha-response"
).innerText='2122988149';
""")
Then you can submit the captcha response, and recaptcha will be solved.
Note: there is a high possibility these steps won’t work. if you are looking for a direct method, you can find it here